- August 28, 2021
- Posted by: Orin Swanston
- Categories: PDF, PHP
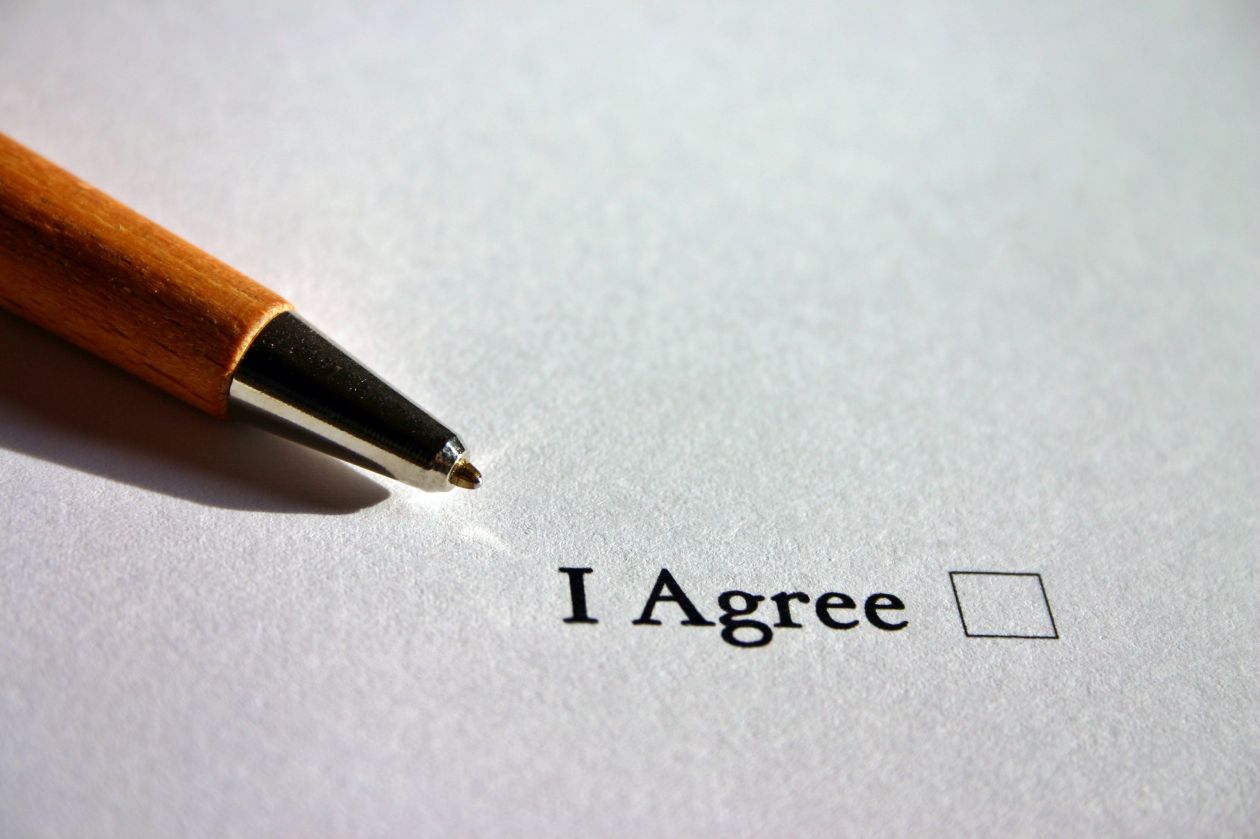
In some cases, we may need to create fillable PDF forms dynamically based on known data that we need to capture. These PDF forms can be saved offline to be completed and printed or saved and submitted as a later time. Forms can also be distributed by email or from a server.
This example requires having composer installed to manage your project libraries
First, let’s start by installing the TCPDF library into your project directory with composer
composer require tecnickcom/tcpdf
In this example, we will be creating a simple form that will allow a user to enter their name and a type of car.
// Load autoloader (using Composer)
require __DIR__ . '/vendor/autoload.php';
$pdf = new TCPDF(); // create TCPDF object with default constructor args
/*TCPDF adds a line to the top and bottom by default. Let's turn those off*/
$pdf->setPrintHeader(false);
$pdf->setPrintFooter(false);
$pdf->AddPage();
Now we add the code that will actually create the input fields.
// First name
$pdf->Cell(35, 5, 'First name:');
$pdf->TextField('firstname', 50, 5);
$pdf->Ln(6);
// Last Name
$pdf->Cell(35, 5, 'Last name:');
$pdf->TextField('lastname', 50, 5);
$pdf->Ln(6);
Since we want the user to be able to choose which car they are driving from a fixed list, we can also use the following code to create a radio button list
// Vehicle
$pdf->Cell(35, 5, 'Vehicle:');
$pdf->RadioButton('vehicle', 5, array(), array(), 'Water');
$pdf->Cell(35, 5, 'Toyota');
$pdf->Ln(6);
$pdf->Cell(35, 5, '');
$pdf->RadioButton('vehicle', 5, array(), array(), 'Beer');
$pdf->Cell(35, 5, 'Ford');
$pdf->Ln(6);
$pdf->Cell(35, 5, '');
$pdf->RadioButton('vehicle', 5, array(), array(), 'Wine');
$pdf->Cell(35, 5, 'Kia');
Lastly, we call the output action to output the pdf to the browser window.
$pdf->Output('out.pdf'); // send the file inline to the browser (default).
And that’s it! Run the example in a browser window to see the result. The PDF should resemble the image below.
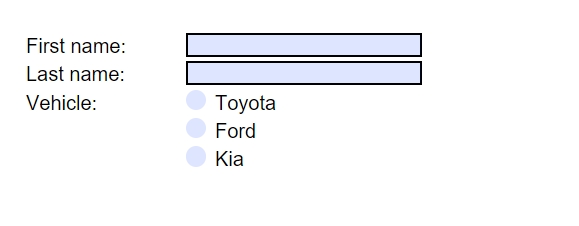