- July 5, 2023
- Posted by: Editor
- Categories: PHP, SharePoint, WordPress
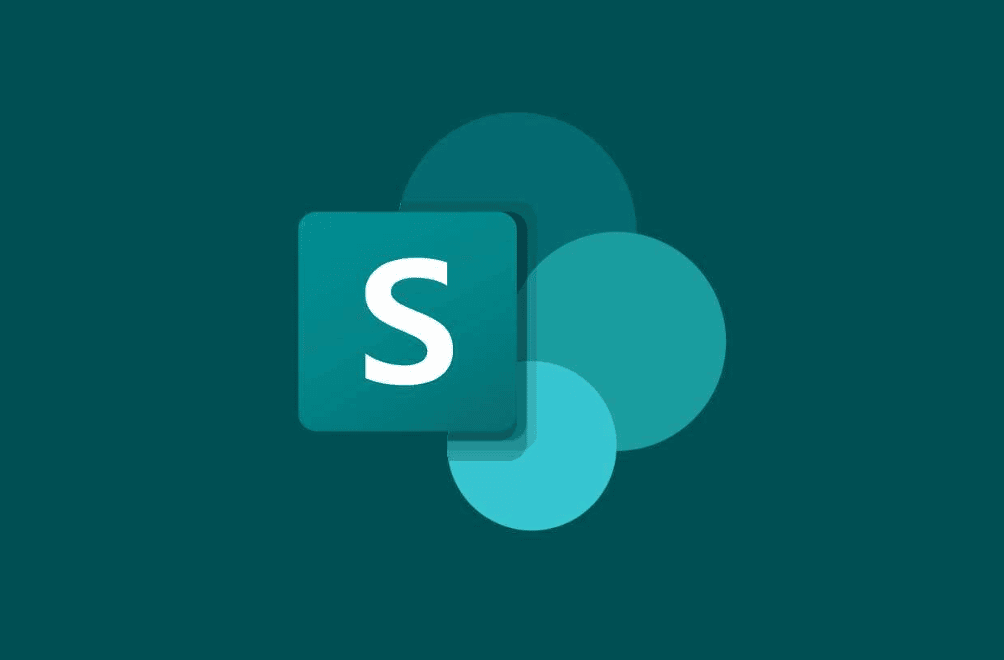
If you work in a hybrid tech environment, you probably work within many different software ecosystems. Getting these applications to talk to each other often isn’t always straightforward but it’s gotten better as many applications now offer ways of communicating with each other using REST APIs or various endpoint for authentication and exchanging information. Today we’re going to be looking at Microsoft SharePoint which is a well-known platform for collaboration and document management. In this article we are going to explore how to download files from a SharePoint instance.
Prerequisites:
Before we get started, ensure you have the following in place:
- A SharePoint site URL where the file is hosted.
- A SharePoint username and password with sufficient permissions to access the file.
Establishing the SharePoint Connection
To download a file from SharePoint, we need to establish a connection and authenticate ourselves. We will use the SharePoint site URL, username and password for this purpose. The Authorization header is set using the base64-encoded username and password.
<?php
$username = 'sharepointuser@mycompany.com';
$password = 'mypasswordhere';
// Set the authentication headers
$authHeaders = [
'Authorization: Basic ' . base64_encode($username . ':' . $password),
];
// Create the stream context with the authentication headers
$context = stream_context_create([
'http' => [
'header' => $authHeaders,
],
]);
Building the File Download URL
Now we construct the file download URL by appending the SharePoint site URL with the relative file path. This URL will be used to download the file using the ‘file_get_contents‘ function. For example if our URL is: ‘https://sharepoint.mycompany.com’ and our relative file path is ‘Documents/sample_document.pdf‘ our file download URL will be https://sharepoint.mycompany.com/Documents/sample_document.pdf
<?php
$source = 'sample_document.pdf';
// SharePoint site URL and file URL
$webUrl = "https://sharepoint.mycompany.com";
$fileUrl = "/Documents".$source;
//optionally replace spaces in $fileUrl
$fileUrl = str_replace(' ', '%20', $fileUrl);
// Create the SharePoint file URL
$fileDownloadUrl = $webUrl . $fileUrl;
To download the file, we can now use the ‘file_get_contents‘ function, passing in the file download URL and a stream context along with the authentication headers. The file content will be fetched and stored in a variable. Be care to ensure that you correctly handle any potential download errors or exceptions such as a file not being found before attempting to save the file to a local directory.
To save the file locally we can use the ‘file_put_contents‘ function to save the downloaded file to a local file. Simply specify the local file path where you would like the downloaded file to be stored. The function will then write the content to the specified directory or path. Optionally we can stream the contents of the file for PDF documents so that they can be rendered directly in the browser itself. If we store the document locally, we can now also modify it or email the document elsewhere entirely.
$fileName = basename($source); //grab the name of the file for download purposes
@$data = file_get_contents($fileDownloadUrl, false, $context);
if(!$data) {
echo 'No document could be found with that name.';
exit;
}
file_put_contents($fileName, $data);
//can also optionally open document in browser if it is a PDF
header('Content-type: application/pdf');
header('Content-Disposition: inline; filename="'.$fileName.'"');
header('Content-Transfer-Encoding: binary');
header('Accept-Ranges: bytes');
echo $data;
exit;
?>
As you can see, we are able to do this without using any third-party libraries by simply leveraging built in PHP functions to establish a connection, authenticate, retrieve the file content and store it locally for further processing. Remember to ensure the security and confidentiality of the SharePoint credentials used to access the instance. These should not be stored directly in the file but elsewhere in a database or other secure location or typed in at the point of when a download is required.
Happy coding!