- August 20, 2021
- Posted by: Orin Swanston
- Category: PHP
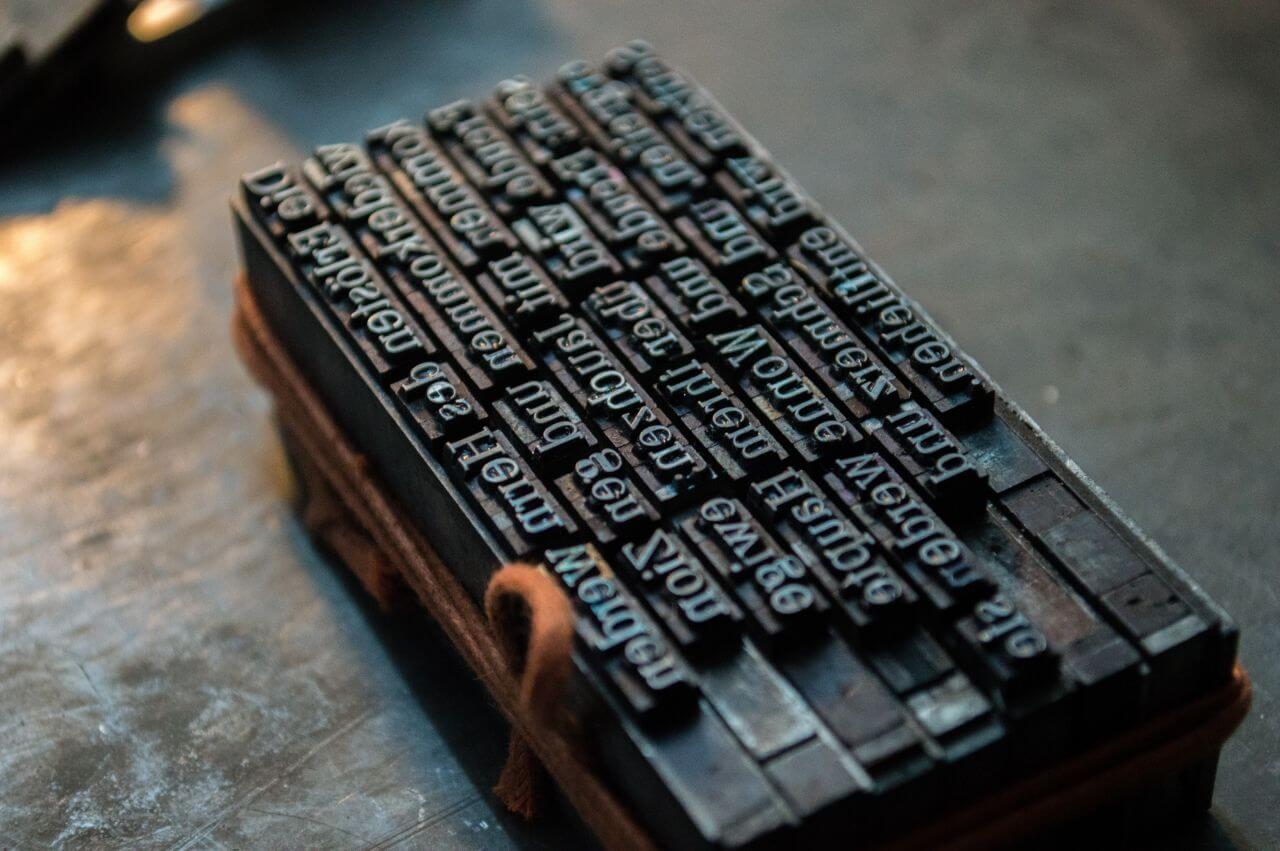
Sometimes you need to create a PDF from scratch rather than just add text to an existing one.
Dompdf is a fantastic tool that quickly and easily converts HTML into PDF. Dompdf is not especially fault tolerant so ensure that your HTML is well-formed before trying to render.
To install with Composer enter the following into your terminal, shell or command line inside your project folder
composer require dompdf/dompdf
Then ensure it’s loaded using the autoload in your main project code
require 'vendor/autoload.php';
Optionally you can download and install instead from the project releases page here and use the packaged autoloader to load the necessary libraries and functions
require_once 'dompdf/autoload.inc.php';
Now you can test your HTML to PDF functionality.
For this example, we’re going to print a student completion certificate.
First we need to create the HTML. You can enhance your HTML using CSS rules inline or embedded as <style></style>. In the example below, we pre-populated the student information so that the results can be displayed within the HTML. You can see how this can be further enhanced by fetching results from a database or other data source to fetch and print student records.
$student = 'John Smith';
$coursename = 'Programming 101';
$points = 95;
$date = date('F m,Y');
$html = "<div style='width:800px; height:600px; padding:20px; text-align:center; border: 10px solid #187bcd;margin:0 auto'>
<div style='width:750px; height:550px; padding:20px; text-align:center; border: 5px solid #187bcd'>
<span style='font-size:50px; font-weight:bold'>Certificate of Completion</span>
<br><br>
<span style='font-size:25px'><i>This is to certify that</i></span>
<br><br>
<span style='font-size:30px'><b>$student</b></span><br/><br/>
<span style='font-size:25px'><i>has completed the course</i></span> <br/><br/>
<span style='font-size:30px'>$coursename</span> <br/><br/>
<span style='font-size:20px'>with score of <b>$points</b></span> <br/><br/><br/><br/>
<span style='font-size:25px'><i>dated</i></span><br>$date
</div>
</div>";
Now we set up the PDF and pass in the HTML that we created previously
// reference the Dompdf namespace
use Dompdf\Dompdf;
// first instantiate and use the dompdf class
$dompdf = new Dompdf();
$dompdf->loadHtml($html);
// (Optional) Setup the paper size and orientation
$dompdf->setPaper('A4', 'landscape');
// Render the HTML as PDF
$dompdf->render();
// Output the generated PDF for download
$dompdf->stream();
If you prefer to display the PDF directly in the browser instead of prompting for download, you can use the following instead.
$dompdf->stream("out.pdf", array("Attachment" => false));
If you want to save the document to file instead of streaming directly to the browser you can save the contents instead using the following. You can amend the file name such as appending the student name, a unique code or date stamp to avoid overwriting the previous save.
$pdfFile = 'out.pdf';
file_put_contents($pdfFile,$dompdf->output());
And that’s it! Now try it on your own and see what other uses you can come up with.